How to declare an object in Objective-C
Creating a new object in Objective-C is usually a two-step process. First, memory has to be allocated for the object, then the object is initialized with proper values. The first step is accomplished through the alloc class method, inherited from NSObject and rarely, if ever, overridden.
How do you define an object in Objective-C?
The instance variables of the class are declared within curly braces { }. The properties and methods of the class are declared after the instance variable declaration. An object is an instance of a class. It is created by allocating memory for it using the alloc method and initializing it using the init method.
Cached
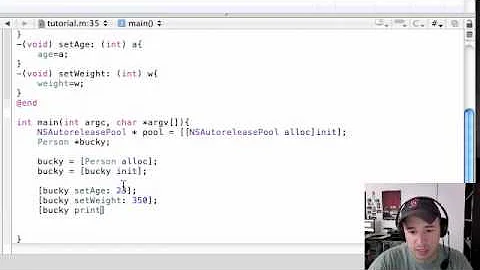
How to initialize an object in Objective-C?
Out of the box in Objective-C you can initialize an instance of a class by calling alloc and init on it. // Creating an instance of Party Party *party = [[Party alloc] init]; Alloc allocates memory for the instance, and init gives it's instance variables it's initial values.
How to create an object of a class in Objective-C?
You can create an instance in Objective C in 3 different ways:
- NOTE. Add the line #import "Product. …
- OR. Product *product = [[Product alloc]init]; //Allocating and initializing an instance of Product class. …
- OR. Product *product = [Product new]; //Allocating and initializing an instance of Product class using 'new' keyword.
Cached
How to declare a method in Objective-C?
An Objective-C method declaration includes the parameters as part of its name, using colons, like this: — (void)someMethodWithValue:(SomeType)value; As with the return type, the parameter type is specified in parentheses, just like a standard C type-cast.
How to define an object?
The Oxford Learner's Dictionary defines an object as “a noun, noun phrase or pronoun that refers to a person or thing that is affected by the action of the verb (called the direct object), or that the action is done to or for (called the indirect object)”.
How do we describe an object?
There are several things we can talk about to describe objects. We can describe the items appearance by describing its size shape.
How to initialize an object?
An object initializer is a comma-delimited list of zero or more pairs of property names and associated values of an object, enclosed in curly braces ( {} ). Objects can also be initialized using Object.create() or by invoking a constructor function with the new operator.
How do you declare and initialize an object?
To create an object of a named class by using an object initializer
- Begin the declaration as if you planned to use a constructor. …
- Type the keyword With , followed by an initialization list in braces. …
- In the initialization list, include each property that you want to initialize and assign an initial value to it.
How do you create a new object in a class?
To create an object of Main , specify the class name, followed by the object name, and use the keyword new :
- Example. Create an object called " myObj " and print the value of x: public class Main { int x = 5; public static void main(String[] args) { Main myObj = new Main(); System. …
- Example. …
- Second.java.
How do you assign an object to a class?
Assigning an Object to Classes
- In the Classification menu, choose Assignment Assign Object to Classes .
- Enter the object you want to classify. …
- Enter the class or classes to which you want to assign your object. …
- If you want to assign values to characteristics, position the cursor on a class.
How do you declare an object class?
Declaring a Variable to Refer to an Object
- The declared type matches the class of the object: MyClass myObject = new MyClass();
- The declared type is a parent class of the object's class: MyParent myObject = new MyClass();
- The declared type is an interface which the object's class implements:
How do you declare an object in oops?
In object-oriented programming, objects are created from classes. To create an object, first, a class is defined with the desired properties and behaviors. Then, an instance of the class is created using the "new" keyword, which allocates memory for the object and initializes its properties.
How to create an object?
Creating an Object
In Java, the new keyword is used to create new objects. Declaration − A variable declaration with a variable name with an object type. Instantiation − The 'new' keyword is used to create the object. Initialization − The 'new' keyword is followed by a call to a constructor.
How do you define an object class?
Object-Oriented Terminology
class: a class describes the contents of the objects that belong to it: it describes an aggregate of data fields (called instance variables), and defines the operations (called methods). object: an object is an element (or instance) of a class; objects have the behaviors of their class.
How is an object identified?
An object can be identified based on the following characteristics: Color: Colour and appearance help to differentiate and classify an object. Texture: Every object possesses a different kind of texture. Thus, it is essential to understand the surface and feel of the object.
How do you define an object in a sentence?
The object of a sentence is the person or thing that receives the action of the verb. It is the who or what that the subject does something to.
How will you declare an object with example?
To create an object of Main , specify the class name, followed by the object name, and use the keyword new :
- Example. Create an object called " myObj " and print the value of x: public class Main { int x = 5; public static void main(String[] args) { Main myObj = new Main(); System. …
- Example. …
- Second.java.
How do you initialize an object class?
There are two ways to initialize a class object:
- Using a parenthesized expression list. The compiler calls the constructor of the class using this list as the constructor's argument list.
- Using a single initialization value and the = operator.
How do you declare an object?
Declaring an Object
Declarations simply notify the compiler that you will be using name to refer to a variable whose type is type. Declarations do not instantiate objects. To instantiate a Date object, or any other object, use the new operator.
How do you declare an object variable?
You use a normal declaration statement to declare an object variable. For the data type, you specify either Object (that is, the Object Data Type) or a more specific class from which the object is to be created. Declaring a variable as Object is the same as declaring it as System.
Can we create object inside class?
Yes, in Java it is possible to create an object of a class inside the same class. This is known as creating an instance of the class within the class.
How do you assign an object?
JavaScript Demo: Object.assign()
- const target = { a: 1, b: 2 };
- const source = { b: 4, c: 5 };
- const returnedTarget = Object. assign(target, source);
- console. log(target);
- console. log(returnedTarget === target);
Can we declare object inside class?
Yes, of course. Officially this is called ' Call By Reference'. You can create an object of a class in any class may it be same or any other class.
How do you declare a class in a class?
In general, class declarations can include these components, in order:
- Modifiers such as public, private, and a number of others that you will encounter later. …
- The class name, with the initial letter capitalized by convention.
- The name of the class's parent (superclass), if any, preceded by the keyword extends.
How do you declare and create an object?
Creating an Object
- Declaration − A variable declaration with a variable name with an object type.
- Instantiation − The 'new' keyword is used to create the object.
- Initialization − The 'new' keyword is followed by a call to a constructor. This call initializes the new object.